A Developer’s Guide to API Filtering: What It Is, How It Works, and Why It Matters
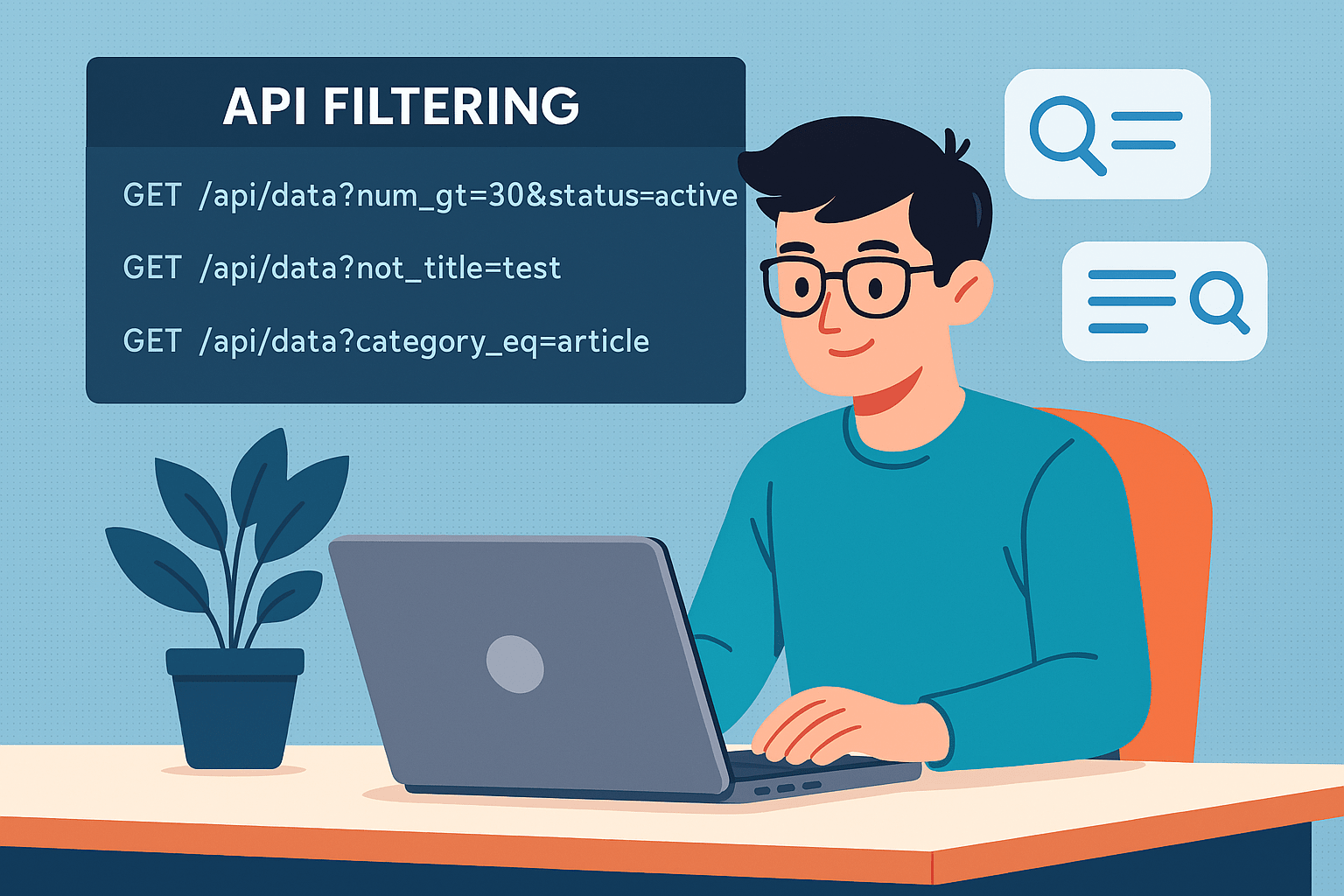
APIs are everywhere. Whether you're working on a web app, a mobile app, or a data platform, chances are you're using an API—or building one. And if you're dealing with APIs, you're definitely dealing with data. But here's the kicker: not all data is useful all the time.
Enter filtering—the humble yet powerful tool that helps you narrow down results, extract meaningful slices of data, and build truly dynamic experiences. In this blog post, we’re going deep into API filtering. We’ll explain what it is, the most common filtering styles, how to choose the right one, and why it matters for clean, scalable architecture.
Grab your ☕ and let’s dive in.
1. What is API Filtering?
Imagine you're browsing an online bookstore with millions of books. If there were no filters, you’d be scrolling endlessly. API filtering is like the search bar and filters that let you say, “Show me all science fiction books written after 2010 by authors whose names start with ‘A’.”
From a technical standpoint, filtering lets clients send specific conditions to the API—usually through query parameters—so they get back only the data that matches.
Why filtering is essential:
- ✅ Improves performance by reducing data payload
- ✅ Gives users control over what they want to see
- ✅ Enables dynamic, responsive interfaces
Whether you're building a basic CRUD API or a complex search engine, filtering makes your data useful, relevant, and fast.
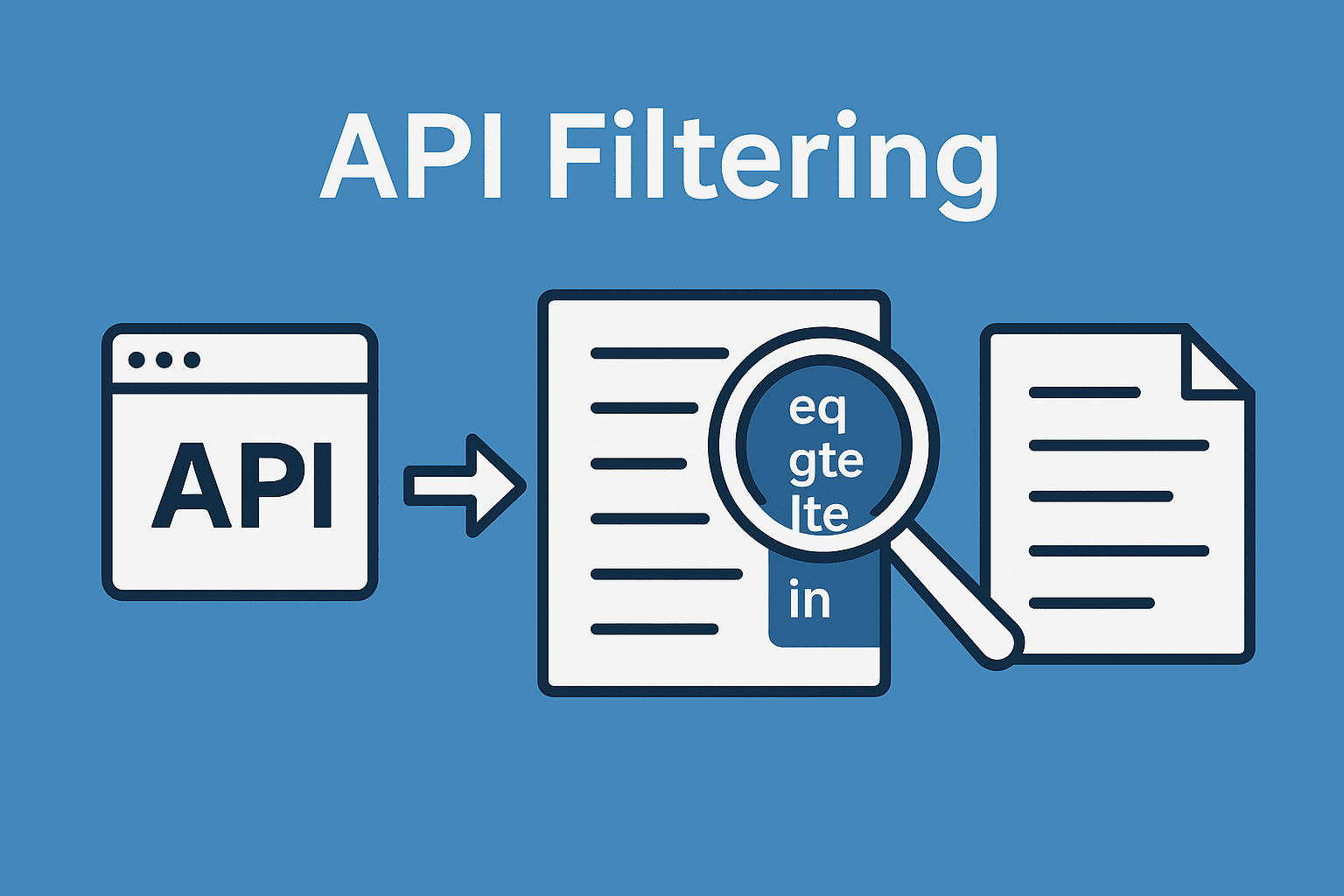
2. Common Filtering Styles (And Their Pros & Cons)
Let’s go beyond bullet points and dive into how each filtering style really works. We'll look at practical use cases, trade-offs, and even sprinkle in a few developer headaches you might want to avoid.
a. Simple Key-Value Filters
GET /api/v1/items?status=active&category=books
This is the vanilla ice cream of filtering. You match fields directly with values. It’s clean, intuitive, and widely supported.
In practice: This style is perfect when you want to expose basic filters in a dashboard. Think of filtering users by status, or blog posts by category. It maps naturally to form fields and UI controls.
Behind the scenes: On the backend, these are easy to parse into SQL WHERE
clauses or ORM filters. You don’t need custom logic—just a bit of string-to-field matching.
But this simplicity comes with a cost.
Drawbacks: You can’t do anything more expressive. No filtering by date ranges. No negation. Want to filter where price > 100
? Too bad.
When it shines: Admin panels, mobile APIs, anywhere low complexity is key.
b. Field Operators (_eq
, _gt
, _lt
, etc.)
GET /api/v1/users?age_gt=25&status_ne=inactive
This is where things start getting spicy. By adding operators to your keys, you unlock comparisons and conditional logic. It’s a favorite among APIs inspired by MongoDB or Elasticsearch.
In practice: You’re no longer limited to checking equality. With _gt
, _lt
, _ne
, and more, you can support filters like "users older than 25", "orders placed before Jan 1", or "accounts not equal to 'banned'".
Behind the scenes: These filters often map to ORM query builder functions or SQL clauses. But parsing becomes trickier—you now need to break up keys like age_gt
into field and operator. Validation also needs to check if the operator is allowed for a given field.
Gotchas: Not all clients understand these out of the box. If your frontend team loves using tools like Axios or Fetch, make sure your documentation clearly shows examples.
Also, naming conventions vary—some APIs use colons (age:gt=25
), some use brackets (age[gt]=25
). Pick a pattern and stick to it like your dev life depends on it.
When it shines: Reports, analytics dashboards, admin tools with date and score ranges.
c. List-Based and Logical Filters
GET /api/v1/products?category_in=[books,electronics]&rating_gte=4
Need to filter by multiple values? Or combine filters with AND
, OR
, and NOT
logic? Welcome to the list-based club.
In practice: This is essential for any UI with checkboxes or multi-select dropdowns. Imagine a product filter where users pick multiple categories, brands, or tags.
Under the hood: You’ll need to support value parsing—often as JSON arrays or comma-separated strings—and handle them in your query logic. This adds complexity, especially when users combine IN
, NOT IN
, and comparison filters.
Some APIs support logical wrappers:
{
"or": [
{"status": "active"},
{"rating_gte": 4.5}
]
}
These are usually passed in POST requests or as part of a search body, especially when URLs get too long or complicated.
Challenges: Encoding can be messy. Users must know whether to use brackets, quotes, or escape characters. Parsing and validating becomes more advanced.
When it shines:
- Dashboards with many filter combos
- Search platforms and e-commerce APIs
- Internal tools that allow data slicing and dicing
d. Query String DSL (Search Syntax)
GET /api/v1/search?q=title:"San Francisco" AND status:active
This one’s for the power users. Query string DSL (domain-specific language) turns your filters into a mini search engine.
In practice: Think Elasticsearch, Facebook Ads rules, or Twitter’s advanced search. Users can combine fields, apply operators, and perform full-text search in a single query string.
Behind the scenes: You need a parser—yes, like a mini language interpreter—to read, validate, and execute these queries. This adds a lot of flexibility, but also risk. Syntax errors, injection attacks, and performance costs need to be handled carefully.
UX trade-off: Great for developers, but intimidating for non-technical users. This isn’t for marketing teams or interns—it’s for APIs where control and power are expected.
When it shines:
- Advanced search interfaces
- Developer platforms and analytics engines
- Tools where filtering logic gets saved, reused, or automated
And hey, if your users ask for nested boolean logic, DSL may be your only sane option.
3. When and Why to Use Each Style
Choosing the right filtering style is less about picking what's most powerful and more about picking what fits your users and your context. Think of it like choosing shoes: running shoes for a marathon, slippers for the couch, and steel-toe boots for a construction site. Same logic applies here.
Let’s walk through some realistic use cases, and explore not just which style to pick—but why it matters.
🛠 CRUD Admin Dashboards
When you’re building internal tools or admin panels, simplicity is king. Most of the time, users are filtering by basic things like status
, role
, or created_at
. Simple key-value filters work beautifully here. No extra logic needed, and your frontend team will thank you.
Example:
GET /api/v1/users?status=active&role=admin
Why overcomplicate when a basic filter does the job?
📊 Analytics or Metrics Dashboards
Things get more interesting when users want to slice data by ranges, group by time, or exclude certain values. This is where field operators (_gt
, _lt
, _ne
) and list filters (_in
) shine. They allow rich, flexible queries while staying readable.
Example:
GET /api/v1/sales?amount_gt=1000®ion_in=["us-east","us-west"]
These styles empower analysts and power users to explore data deeply—without waiting for new endpoints to be built.
🔍 Search Tools and Developer APIs
When search is a core feature—think documentation search, code repositories, or e-commerce—you’ll likely need more expressive filtering. A DSL-style query allows compound logic, text search, and nested conditions.
Example:
GET /api/v1/search?q=category:electronics AND price:[100 TO 500] AND NOT brand:"Acme"
Is it harder to implement? Yes. Does it pay off for the right audience? Absolutely.
📈 Internal Business Intelligence (BI) Tools
BI platforms are usually used by analysts who want flexibility. These tools benefit from a hybrid approach: combining list filters, range queries, and boolean logic.
Example:
{
"and": [
{"signup_date_gte": "2023-01-01"},
{"plan_in": ["pro", "enterprise"]},
{"churned": false}
]
}
This approach is also future-proof—easy to extend, save, and reuse.
📱 Mobile APIs (Performance-Sensitive Environments)
Mobile apps have limited bandwidth and slower connections. Filtering here should be as lean and server-driven as possible. Use simple key-value filters or predefined filters mapped to backend logic.
Example:
GET /api/v1/feed?filter=trending
Avoid sending deeply nested filters over the wire. You’ll save on payload, reduce parsing errors, and improve app speed.
Your Filtering Cheat Sheet
Use Case | Recommended Filter Style |
---|---|
Admin dashboard | Simple key-value |
Analytics/reporting | Field operators + list filters |
Search interface | DSL-style q= filters |
Internal BI tools | Combination of logical + range filters |
Mobile APIs | Key-value with server-side defaults |
Pro Tips:
- Start with the simplest solution and iterate as user needs grow.
- Think from the client’s perspective—what data do they really need?
- Keep naming consistent across filters. Predictability = joy 🧘
- Document edge cases and error responses. Filtering can get weird fast.
Filters may seem like a boring part of API design, but they’re the gateway to almost every dynamic experience. Treat them with care—and your users will fly through your data like butter on a hot pancake.
4. The Role of Filtering in API Design & Architecture
Filtering isn’t just a helpful feature—it’s a foundational design decision. It affects how clients consume your data, how your backend performs, and how easy it is to extend your system in the future. Let’s unpack why filtering deserves serious architectural attention.
🧼 Clean Data Interfaces
Imagine an API that returns everything by default: every record, every field, every relationship. It’s like ordering a coffee and receiving every drink on the menu. Overwhelming, slow, and wasteful.
Well-designed filters let clients request exactly what they need—nothing more, nothing less. This not only simplifies client code but also ensures clean, focused communication between client and server.
"Give me all active users" becomes?status=active
. "Show blog posts tagged with 'AI'" becomes?tags_in=ai
.
⚙️ Scalable and Performant Backend
Filtering at the database level reduces the amount of data fetched, serialized, and sent over the wire. This matters more as your dataset grows.
A few examples:
- Without filters:
SELECT * FROM users;
😬 - With filters:
SELECT * FROM users WHERE status = 'active';
✅
Now multiply that across services, microservices, and client requests—it adds up. Filtering early (preferably at the DB or index layer) means your backend stays snappy under load.
🔌 Decoupled and Flexible Client Development
When you expose a versatile filtering system, your API becomes a toolkit. Frontend teams, mobile apps, and even third-party developers can build features without waiting on backend changes.
Instead of asking, “Can you add a new endpoint for users who signed up this month?”, they can just use:
GET /api/v1/users?signup_date_gte=2024-03-01
This decouples frontend/backend development and boosts velocity for everyone.
🏗 Future-Proof Extensibility
You might start with status
and category
, but one day your app may need:
- ML prediction confidence filters
- Event-based date filters
- Real-time metrics thresholds
With a consistent filtering foundation (think: operator support, field whitelisting, structured parsing), you can evolve your system without rework or rewrites.
Think of filters as the query language of your API. Get it right early, and you’ll build something your team and users love.
Bonus tip: Avoid hardcoding logic per endpoint. Centralize parsing, validation, and translation into query logic. This saves your bacon when complexity ramps up.
🔄 Consistency Across Endpoints
Consistency makes APIs easier to learn and easier to scale. If ?status=active
works on /users
, it should behave the same on /orders
, /sessions
, and beyond. This predictability breeds developer trust.
When filters behave differently depending on the endpoint, confusion sets in—and you’ll spend more time writing docs and debugging misunderstandings than building features.
So unify your filtering logic. Design once, apply everywhere. Like reusable Lego blocks, but for data!
Filtering might seem small, but it punches way above its weight in API design. Whether you go simple or advanced, the key is to choose what fits your product and your users. Build it well, document it clearly, and make it a joy to use.
Want to learn how big platforms like Facebook, Twitter, and 2600hz design their filters? Check out the links below!
Member discussion